Well, as you all know Unity team has recently released Unity version 4.5 which brought us (according to Unity's web site):
- Over 450 bug fixes,
- OpenGL ES 3.0,
- Shader workflow boost,
- Smooth and natural 2D physics and
- Sparse Textures.
While 450 bugs fixed sounds amazing, shaders compilation was indeed terribly slow, I don't really care about OpenGL ES 3.0 (since I got an old iPhone 4s) and Sparse Textures is a good feature to explore on modern desktop GPUs, what I want to tell you about are those features and bug fixes buried in the massive Release Notes which you have probably missed but they are indeed very important.
In no particular order.
Hierarchy Window sorting
New Hierarchy Window sorting - sorting of elements is now based on transform order instead of name.
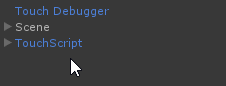
This change alone breaks the naming convention of millions of developers. I myself used to name the empty object with controllers $ to make sure that it's always on the top of Hierarchy Panel. Now you can just drag GameObjects up and down manually.
SelectionBase attribute
Editor: Created SelectionBase attribute. When applied to a script, GameObjects with that script on will work the same as prefab roots for Scene View picking.
If you have an hierarchy of objects with geometry attached usually when you click one of them it gets selected.
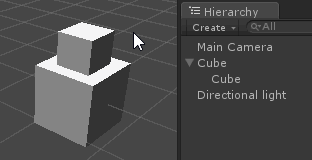
If you remember Prefabs behave differently. If you click an object in prefab's hierarchy the root of this prefab is be selected instead. And now you can manually force this behavior using SelectionBase attribute.
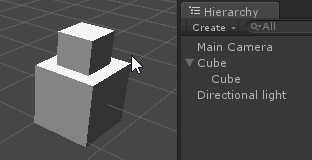
[SelectionBase]
public class TheBase : MonoBehaviour {}
This may be useful when you think of an hierarchy of objects as single object and don't want to control its child objects. For example you have an object and a plane inside and it's important to move this root object instead of its child plane. This feature might help you always select the right object.
DecoratorDrawers
Editor: PropertyDrawers are now supplemented with DecoratorDrawers, which can be used for decorative elements in the Inspector such as spacing, headers, or notes.
Editor: Improved inspector customisation with [Tooltip] and [ContextMenuItem] script attributes.
PropertyDrawers are used to make your fields more pretty in the Editor. They are extremely easy to use. For example using just one annotation you can convert a float field into a smart range property.
[Range(0, 100)]
public float MyValue;
While PropertyDrawers modify the appearence of fields in the Editor, new DecoratorDrawers augment them with visual elements. Check out this code:
[Header("Hi there!")]
public string TheHeader = "Header!";
[Tooltip("This is THE VALUE!")]
[ContextMenuItem("Reset", "resetTheValue")]
public float TheValue = 42.0f;
[Space(50)]
public string TheString = "THE STRING";
private void resetTheValue()
{
TheValue = 42;
}
And the animation where you can see what these annotations do.
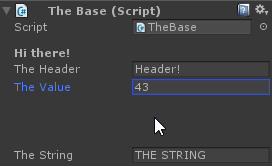
Just a little trick to make your Editor interfaces better.
Scripts in DLLs
Scripting: Improved resolution of types that inherit from types in different user assemblies.
This is probably one of my favorites.
You see, prior to 4.5 if you had MyBaseClass : MonoBehaviour
in MyBaseClass.dll and MyDerivedClass : MyBaseClass
in MyDerivedClass.dll you couldn't use MyDerivedClass in your projects because Unity was unable to follow this MASSIVE inheritance chain and figure out that MyDerivedClass was a MonoBehaviour.
Thanks God this is now fixed.
Default parameters
Scripting: Fixed namespace detection for MonoBehaviors containing methods with default parameters.
This bug was one of those bugs stopping people from using specific language features. Now we can breath freely.
Saving to JPEG
Graphics: Added Texture2D.EncodeToJPG.
Many developers have been asking for a function to save to JPEG. Yes we had Texture2D.EncodeToPNG for a long time, but you know...
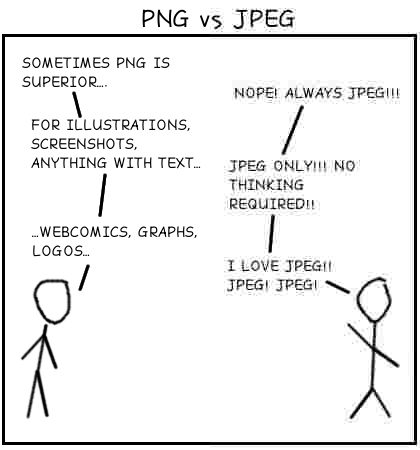
Anyway, the new method is also terribly slow. Encoding a 2048x2048 texture:
- Encoded to PNG in 0.4529677 seconds,
- Encoded to JPG in 0.1349338 seconds.
Now we know if we have Pro license
Scripting: Added UNITY_PRO_LICENSE define.
This will be useful for fellow plugin developers. Now we have an easy way to be sure that code we are writing targets right Editor version.
Serialization
Scripting: Add UnityEngine.ISerializationCallbackReceiver interface, to get a callback right before serialisation and right after deserialization.
Scripting: Structs with System.Serializable attribute can now be serialized. Also, fields of AnimationCurve[] and double[] now get serialised.
People have been asking for years for the ability to serialize structs. Now this code works and shows a list of structs:
public List<MyStruct> list = new List<MyStruct>();
[Serializable]
public struct MyStruct
{
public int Value;
}
Being able to serialize arrays of AnimationCurve sometimes may be handy too.
But what really interesting is ISerializationCallbackReceiver interface. The docs say:
Interface to receive callbacks upon serialization and deserialization.
It seems that instead of wasting man-years on figuring out how to serialize Dictionaries they gave us an ability to manually specify what and how we want to be serialized. The example in the docs shows just that.
GameObject.GetComponentsInParent()
Scripting: Added GetComponentsInParent function.
It's good to know that now we have this. I haven't tested it yet but it must be a lot faster than enumerating manually through the parent chain.
Debugging DirectX 11 shaders
Documentation: Added docs on how to debug DirectX11 shaders with Visual Studio, look for 'SL-Debugging DirectX 11 Shaders with Visual Studio'.
The method described is not new but now we got a decent tutorial on how to debug dx11 shaders in Unity.
Windows Phone apps
I understand that nobody gives a damn about WP apps but I had to develop a couple simple ones for Nokia Lumia and I'm glad that Unity is evolving and I hope that it will be able to make this platform better.
There are a lot of added features and fixed bugs for Windows Phone target and it's good to know that I helped fixing some of them:
Start, Update and similar methods in scripts derived from generic base class are now properly invoked.
WP: Resolution will now be correctly detected on phones than have larger screen than 768x1280.
WP: Screen.dpi now returns physical device DPI, rather than 0.
o.O
Active scenes count properly displayed when more than 100 scenes are added to Build Settings window.
Damn, some people have huge projects...
Nice lists
And the last one is not even in the Release Notes. It's a little Editor class hiding in UnityEditorInternal
namespace. It's called ReorderableList
and it makes drawing lists in custom inspectors such an easy task. If you ever tried to make a pretty inspector for a List<> or Array field in Unity you know what a massive pain it is.
Here's a demo of ReorderableList class:
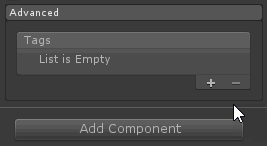
This is the code from the custom inspector used:
private ReorderableList list;
list = new ReorderableList(property.serializedObject, property.FindPropertyRelative("tagList"), true, true, true, true);
list.drawHeaderCallback += rect => GUI.Label(rect, label);
list.drawElementCallback += (rect, index, active, focused) =>
{
rect.height = 16;
rect.y += 2;
EditorGUI.PropertyField(rect,
list.serializedProperty.GetArrayElementAtIndex(index),
GUIContent.none);
};
list.DoList(position);
This is the full example: TouchManagerEditor.cs
Interesting fact: there was already practically identical list implementation called ReorderableList which I've been using from time to time.
***
These are the features I found particularly interesting while browsing through Unity 4.5 Release Notes. It's always like a treasure hunt... you never know what you will find.
If for some reason you think that some other features/fixes deserve more attention feel free to post them in comments.